Figure and Axes
Figure의 instance는 matplotlib가 그리는 그림 하나당 하나가 할당되며, 그림이 그려질 canvas 영역을 제공한다.
- Figure는 1개 이상의 Axes를 가질 수 있음.
Axes의 instance는 Figure가 제공하는 전체 canvas의 특정 고정영역에 할당된 좌표계를 제공한다.
- Axes의 instance는 Figure에 수동으로 삽입될 수도 있지만, matplotlib에서는 여러 layout manager를 사용해 자동으로 추가되기도 한다.
(일반적으로 하나의 method 호출로 Figure와 포함된 Axes를 동시에 얻는 게 일반적임) - matplotlib에서 그래프를 그리는 대부분의 method를 제공하며 axis의 tick과 label 등을 그리고 조절하는 methode들도 Axes의 instance들의 method로 제어됨.
Figure
그래프가 그려지는 canvas 영역을 제공.
다음과 같이 plt.figure
를 사용해 단독으로생성 가능함.
import matplotlib.pyplot as plt
fig = plt.figure(
figsize=(width_inch, height_inch),
facecolor='w')
figsize
: 전체 canvas의 넓이와 높이를 inch의 sequence type으로 받음.facecolor
: canvas의 color을 지정함.
savefig
method를 통해, 특정 format의 image로 저장가능함. matplotlib에서 주로 사용되는 format은 다음과 같음.
- PNG
- SVG
format은 argument로 지정되는 파일경로의 file extension에 의해 자동으로 결정되지만,
foramt
파라메터에 명시적으로 지정할 수 있음.
단, 저장되는 image에서 canvas color를 facecolor
로 다시 지정을 해줘야한다.
또한 transparent=True
로 설정하고 png와 같이 alpha channel을 제공하는 format으로 저장하는 경우 배경이 투명한 image로 저장도 가능함.
...
fig.savefig(
'test.png',
dpi = 150,
facecolor='r'
)
Axes
Figure 의 instance가 제공하는 canvas 영역에 할당되는 Axe의 instance는 해당 위치에서 실제 그래프를 그리는 methods를 제공하는 일종의 entry point 역할을 수행한다.
다음과 같이 Figure의 instance의 add_axes
method를 통해 명시적으로 추가될 수 있다.
left = right = 0.1 # canvas의 upper left를 원점으로 넓이의 10%,높이의 10%.
width = height = 0.8 # Axes가 실제 그리는 영역의 넓이와 폭이 canvas의 80%.
ax = fig.add_axes(
(left, right, width, height),
facecolor='#d8d8d8"
)
...
facecolor
: Axes가 그려지는 영역 전체의 color를 지정함.
Example for Figure and Axes : fig.add_axes
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(-1,1,100)
y0 = 0.5*x+ 0.2
y1 = np.sin(x - 0.5)
fig = plt.figure(figsize=(6,3), facecolor='r')
# fig에 axes 객체를 명시적으로 위치를 지정하여 추가.
left = right = 0.1 # canvas의 upper left를 원점으로 넓이의 10%,높이의 10%.
width = height = 0.8 # Axes가 실제 그리는 영역의 넓이와 폭이 canvas의 80%.
ax = fig.add_axes(
(left, right, width, height),
facecolor='#d8d8d8'
)
ax.plot(x,y0)
ax.plot(x,y2)
fig.savefig('test.png',dpi = 150,facecolor='r')
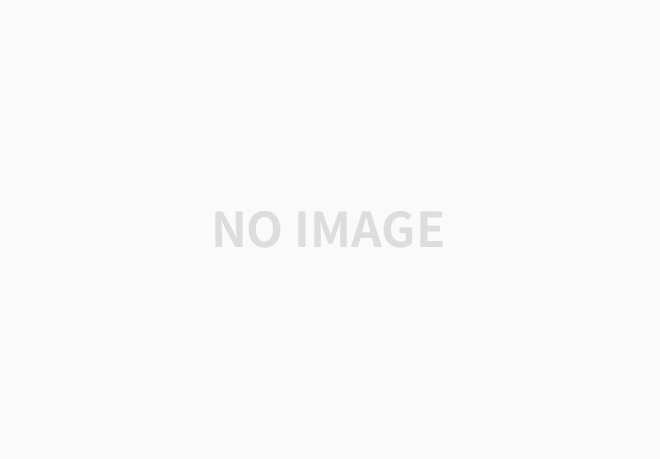
Example for Figure and Axes : plt.subplots
위의 예에서 Axes의 instance를 fig.add_axes
를 이용하여 canvas내의 위치와 크기를 명시적으로 지정했지만, 일반적으로는 그래프의 layout을 관리하는 layout manager를 통해 Figure와 Axes의 인스턴스를 동시에 얻는게 일반적임.
가장 널리 사용되는 방식은 plt.subplots
를 이용한 방식으로 grid layout으로 그리고자 하는 그래프를 배치한다.
- 반환값은 Figure 인스턴스와 Axes 인스턴스들의 collection임.
- 반환된 Axes 인스턴스는
nrows
× ncols
의 shape를 가진 numpy ndarray임.
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(-3,3,100)
y0 = 0.5*x+ 0.2
y1 = np.sin(x - 0.5)
y2 = np.cos(x - 0.5)
y3 = -0.5*x+0.2
fig, axs = plt.subplots(
nrows = 2,
ncols = 2,
figsize = (12,3)
)
axs[0,0].plot(x,y0, label='chart0')
axs[0,1].plot(x,y1, label='chart1')
axs[1,0].plot(x,y2, label='chart2')
axs[1,1].plot(x,y3, label='chart3')
# fig.legend() # 전체 canvase에 모든 subplot들에 대한 legend생성.
axs[0,0].legend() # upper left의 subplot에 대한 legend 생성.
다음은 결과 그래프임.
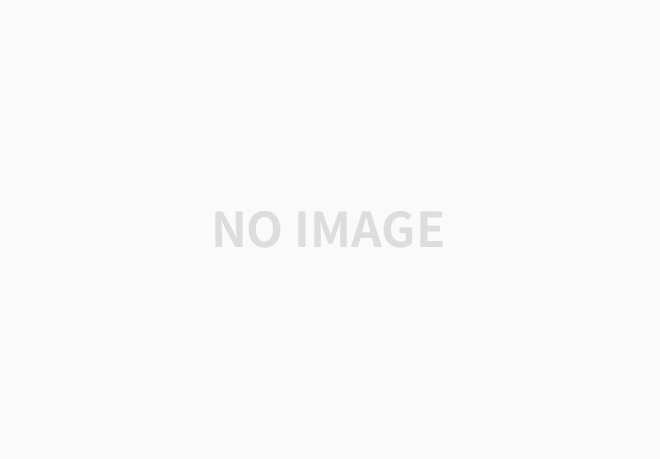
참고로, 좀 더 복잡한 layout을 위해서 Matplotlib는 다음의 layout mangers를 제공함.
2024.01.22 - [Python/matplotlib] - [matplotlib] Layout Managers: GridSpec and subplot2grid
[matplotlib] Layout Managers: GridSpec and subplot2grid
Matplotlib에서 여러 graph에 해당하는 Axes 객체들을 하나의 Figure객체에 포함시킬 경우, 이들을 어떻게 배치할지를 정해줘야 한다. plt.subplots 을 사용할 경우, 행과 열을 지정하지만 모두 같은 크기를
ds31x.tistory.com
관련 gist url
https://gist.github.com/dsaint31x/505437f3885373c392cb30f5558d3e03
py_matplot_fig_axs.ipynb
py_matplot_fig_axs.ipynb. GitHub Gist: instantly share code, notes, and snippets.
gist.github.com
같이 읽어보면 좋은 자료들
2024.06.03 - [Python/matplotlib] - [matplotlib] Summary : 작성중
[matplotlib] Summary : 작성중
Introduction2024.03.04 - [Python/matplotlib] - [matplotlib] matplotlib란 [matplotlib] matplotlib란Matplotlib은 Python에서 가장 널리 사용되는 Data Visualization Library임. matplotlib를 통해 chart(차트), image(이미지) 및, 다양한 vi
ds31x.tistory.com
[Python] matplotlib 의 계층구조 및 Container : Figure, Axes, Axis
matplotlib의 계층구조 matplotlib는 다음과 같은 hierarchical structure를 가지고 있음. 일반적으로 Figure는 하나 이상의 Axes를 가지며(포함하며), Axes는 일반적으로 2개의 Axis 를 포함(2D image인 경우)함. (Axis
ds31x.tistory.com
'Python > matplotlib' 카테고리의 다른 글
[matplotlib] line 및 marker 설정하기. (0) | 2023.07.21 |
---|---|
[matplotlib] : backend 란 (0) | 2023.07.20 |
[Python] matplotlib : Axis Scale and Ticks (0) | 2023.07.14 |
[matplotlib] : Styling Artists and Labeling Plots (0) | 2023.07.14 |
[matplotlib] 계층구조 및 Container : Figure, Axes, Axis (0) | 2023.07.14 |